int
A whole number.
The number can be negative, zero, or positive. As Typst uses 64 bits to store integers, integers cannot be smaller than -9223372036854775808
or larger than 9223372036854775807
.
The number can also be specified as hexadecimal, octal, or binary by starting it with a zero followed by either x
, o
, or b
.
You can convert a value to an integer with this type's constructor.
Example
#(1 + 2) \
#(2 - 5) \
#(3 + 4 < 8)
#0xff \
#0o10 \
#0b1001
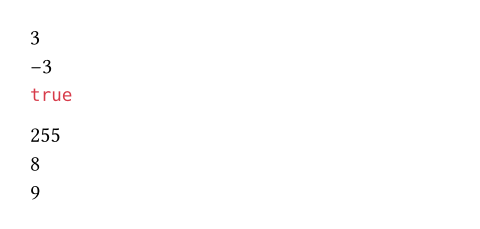
Constructor
Converts a value to an integer.
- Booleans are converted to
0
or1
. - Floats are floored to the next 64-bit integer.
- Strings are parsed in base 10.
#int(false) \
#int(true) \
#int(2.7) \
#(int("27") + int("4"))
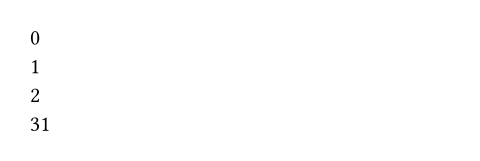
value
The value that should be converted to an integer.
Definitions
signum
Calculates the sign of an integer.
- If the number is positive, returns
1
. - If the number is negative, returns
-1
. - If the number is zero, returns
0
.
#(5).signum() \
#(-5).signum() \
#(0).signum() \
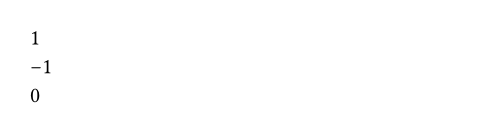
bit-not
Calculates the bitwise NOT of an integer.
For the purposes of this function, the operand is treated as a signed integer of 64 bits.
#4.bit-not()
#(-1).bit-not()

bit-and
Calculates the bitwise AND between two integers.
For the purposes of this function, the operands are treated as signed integers of 64 bits.
#128.bit-and(192)

rhs
The right-hand operand of the bitwise AND.
bit-or
Calculates the bitwise OR between two integers.
For the purposes of this function, the operands are treated as signed integers of 64 bits.
#64.bit-or(32)

rhs
The right-hand operand of the bitwise OR.
bit-xor
Calculates the bitwise XOR between two integers.
For the purposes of this function, the operands are treated as signed integers of 64 bits.
#64.bit-xor(96)

rhs
The right-hand operand of the bitwise XOR.
bit-lshift
Shifts the operand's bits to the left by the specified amount.
For the purposes of this function, the operand is treated as a signed integer of 64 bits. An error will occur if the result is too large to fit in a 64-bit integer.
#33.bit-lshift(2)
#(-1).bit-lshift(3)

shift
The amount of bits to shift. Must not be negative.
bit-rshift
Shifts the operand's bits to the right by the specified amount. Performs an arithmetic shift by default (extends the sign bit to the left, such that negative numbers stay negative), but that can be changed by the logical
parameter.
For the purposes of this function, the operand is treated as a signed integer of 64 bits.
#64.bit-rshift(2)
#(-8).bit-rshift(2)
#(-8).bit-rshift(2, logical: true)

shift
The amount of bits to shift. Must not be negative.
Shifts larger than 63 are allowed and will cause the return value to saturate. For non-negative numbers, the return value saturates at 0
, while, for negative numbers, it saturates at -1
if logical
is set to false
, or 0
if it is true
. This behavior is consistent with just applying this operation multiple times. Therefore, the shift will always succeed.
logical
Toggles whether a logical (unsigned) right shift should be performed instead of arithmetic right shift. If this is true
, negative operands will not preserve their sign bit, and bits which appear to the left after the shift will be 0
. This parameter has no effect on non-negative operands.
Default: false