arguments
Captured arguments to a function.
Argument Sinks
Like built-in functions, custom functions can also take a variable number of arguments. You can specify an argument sink which collects all excess arguments as ..sink
. The resulting sink
value is of the arguments
type. It exposes methods to access the positional and named arguments.
#let format(title, ..authors) = {
let by = authors
.pos()
.join(", ", last: " and ")
[*#title* \ _Written by #by;_]
}
#format("ArtosFlow", "Jane", "Joe")
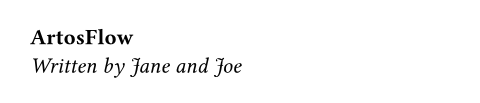
Spreading
Inversely to an argument sink, you can spread arguments, arrays and dictionaries into a function call with the ..spread
operator:
#let array = (2, 3, 5)
#calc.min(..array)
#let dict = (fill: blue)
#text(..dict)[Hello]

Constructor
Construct spreadable arguments in place.
This function behaves like let args(..sink) = sink
.
#let args = arguments(stroke: red, inset: 1em, [Body])
#box(..args)
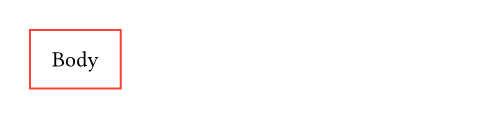
arguments
anyRequiredPositionalVariadic
The arguments to construct.
Definitions
at
Returns the positional argument at the specified index, or the named argument with the specified name.
If the key is an integer, this is equivalent to first calling pos
and then array.at
. If it is a string, this is equivalent to first calling named
and then dictionary.at
.
key
The index or name of the argument to get.
default
any
A default value to return if the key is invalid.
pos
Returns the captured positional arguments as an array.
named
Returns the captured named arguments as a dictionary.